Table of Contents
Create AREA ROOT Class for Shared Memroy Object Area
The first step is to create an Area Rool class. ( let’s say ZCL_ROOT)
Go to SAP Transaction SE24 and create a new class.
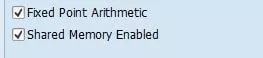
Make sure you checked “Shared Memory Enabled” under Class/Interface Propertiess Tab as following:
Create 2 Instance Attributes
The next step is to create, for example, 2 Instance Attributes within this class.
The Visibility has to be set to Private .
Create SET_DATA & GET_DATA Method
Both of these methods SET_DATA and GET_DATA with handle Shared Memory Object Write and Read operation. We will come back to these methods’ implementation later.
Check alsoHow to use EXPORT or IMPORT data to / from ABAP Memory ID
Create SAP Shared Memory Area ( SHMA )
Launch the Transaction SHMA and create a new Area Name. (ZCL_MEM_AREA for sample)
Fill a short description for the Memory Shared Area. In the Root Class, put the class you have created above ( ZCL_ROOT ).
When saving, SAP will generate an area Class with the same name as the Area Name ( ZCL_MEM_AREA ). The new generated Area Class will contains all the methods needed to read, write and update the Shared Memory Object.
Shared Memory Object Area Class’s Methods
Method | Visibility | Description |
---|---|---|
ATTACH_FOR_READ | Public | Request a Read Lock |
ATTACH_FOR_UPDATE | Public | Request a Change Lock |
ATTACH_FOR_WRITE | Public | Request a Write Lock |
BUILD | Static | Direct Call of Area Constructor |
SET_ROOT | Instanciate | Sets Root Objects |
SET_DATA and GET_DATA Implementation
Once you have created the area root class, the area class and generated the area class, you can implement the ABAP code for the SET_DATA and GET_DATA in order to read/Write the Shard Memory Object.
Write data in Shared Memory Area (SET_DATA)
In order to save data into an instance of Shared Memory Object, you can set the data as following :
DATA: my_handle TYPE REF TO zcl_mem_area. DATA: my_root TYPE REF TO zcl_area_root. TRY . CALL METHOD zcl_mem_area=>attach_for_write EXPORTING inst_name = cl_shm_area=>default_instance attach_mode = cl_shm_area=>attach_mode_default wait_time = 0 RECEIVING handle = my_handle. CREATE OBJECT my_root AREA HANDLE my_handle. CALL METHOD my_root->set_data EXPORTING name = 'name_test' value = 'value_test'. CALL METHOD my_handle->detach_commit. CATCH cx_shm_exclusive_lock_active . CATCH cx_shm_version_limit_exceeded . CATCH cx_shm_change_lock_active . CATCH cx_shm_parameter_error . CATCH cx_shm_pending_lock_removed . ENDTRY.
Read data in Shared Memory Area (GET_DATA)
here an example how to use the GET_DATA in order to read an instance of Shared Memory Object:
DATA: my_handle TYPE REF TO zcl_mem_area. DATA: my_root TYPE REF TO zcl_area_root. TRY . CALL METHOD zcl_mem_area=>attach_for_read EXPORTING inst_name = zcl_mem_area=>attach_for_write RECEIVING handle = my_handle. CREATE OBJECT my_root AREA HANDLE my_handle. CALL METHOD my_root->get_data IMPORTING name = lv_name value = lv_value. CALL METHOD my_handle->detach_commit. CATCH cx_shm_inconsistent . CATCH cx_shm_no_active_version . CATCH cx_shm_read_lock_active . CATCH cx_shm_exclusive_lock_active . CATCH cx_shm_parameter_error . CATCH cx_shm_change_lock_active . ENDTRY.
Note that NAME and VALUE are the attribute of the Area Root Class.
List of Area Root Class Exception
Here the list of the main exception Class for Area Root: